Object3D¶
This module contains all geometric 3D objects which can be added to a scene. All 3D objects inherit from the Object3D base class. 3D objects are defined via a color, the object size, and a name.
Circle¶
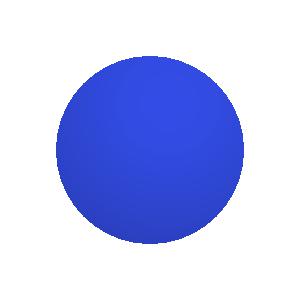
-
class
pysg.object_3d.
CircleObject3D
(radius: float, color=(1, 1, 1), name: str = 'CircleObject')¶ Creates a simple circle geometry.
Note
Per default, only the front face of a plane gets rendered. If you want to also show the backface, you have to configure the moderngl render context. See Renderer section for more information.
Parameters: - radius (float) – Radius of circle.
- color (tuple) – Color of 3D object.
- name (str) – Name of object.
Plane¶
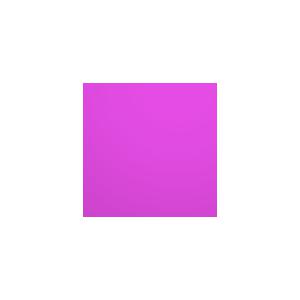
-
class
pysg.object_3d.
PlaneObject3D
(width: float, height: float, color=(1, 1, 1), name: str = 'PlaneObject')¶ Creates a simple plane geometry.
Note
Per default, only the front face of a plane gets rendered. If you want to also show the backface, you have to configure the moderngl render context. See Renderer section for more information.
Parameters: - width (float) – Width of plane.
- height (float) – Height of plane.
- color (tuple) – Color of 3D object.
- name (str) – Name of object.
Triangle¶
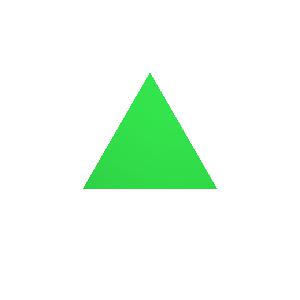
-
class
pysg.object_3d.
TriangleObject3D
(width: float, height: float, color=(1, 1, 1), name: str = 'TriangleObject')¶ Creates a icosahedron geometry.
Parameters: - width (float) – Width of triangle.
- height (float) – Height of triangle.
- color (tuple) – Color of 3D object.
- name (str) – Name of object.
Cube¶
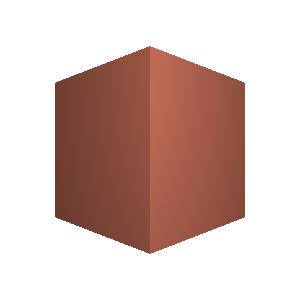
-
class
pysg.object_3d.
CubeObject3D
(width: float, height: float, depth: float, color=(1, 1, 1), name: str = 'CubeObject')¶ Creates a simple cube geometry
Parameters: - width (float) – Width of cube.
- height (float) – Height of cube.
- depth (float) – Depth of cube.
- color (tuple) – Color of 3D object.
- name (str) – Name of object.
Icosahedron¶
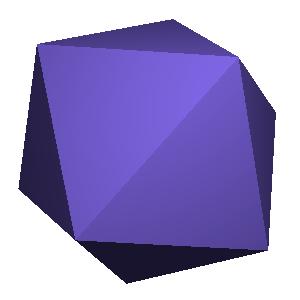
-
class
pysg.object_3d.
IcosahedronObject3D
(radius: float, color=(1, 1, 1), name: str = 'IcosahedronObject')¶ Creates a icosahedron geometry.
Parameters: - radius (float) – Radius of icosahedron.
- color (tuple) – Color of 3D object.
- name (str) – Name of object.
Cylinder¶
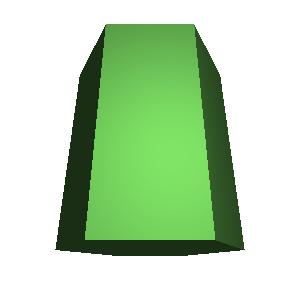
-
class
pysg.object_3d.
CylinderObject3D
(height: float, radius: float, color=(1, 1, 1), name: str = 'CylinderObject')¶ Creates a icosahedron geometry.
Parameters: - width (float) – Width of triangle.
- height (float) – Height of triangle.
- color (tuple) – Color of 3D object.
- name (str) – Name of object.
Tetrahedral¶
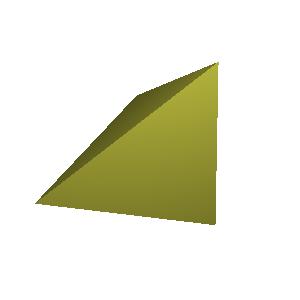
-
class
pysg.object_3d.
TetrahedralObject3D
(radius: float, color=(1, 1, 1), name: str = 'BoxObject')¶ Creates a tetrahedral geometry.
Parameters: - radius (float) – radius of edge points lying on unit sphere.
- color (tuple) – Color of 3D object.
- name (str) – Name of object.
Pyramid¶
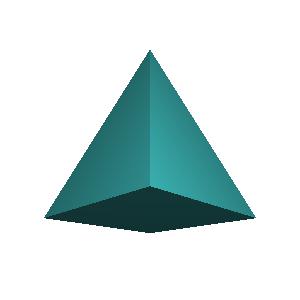
-
class
pysg.object_3d.
PyramidObject3D
(base_size: float, height: float, color=(1, 1, 1), name: str = 'PyramidObject')¶ Creates a square base pyramide geometry.
Parameters: - base_size (float) – Size of the pyramid square base.
- height (float) – Pyramid height.
- color (tuple) – Color of 3D object.
- name (str) – Name of object.